Byte-Sized Tip #1: Navigating Private Keys in Environment Variables
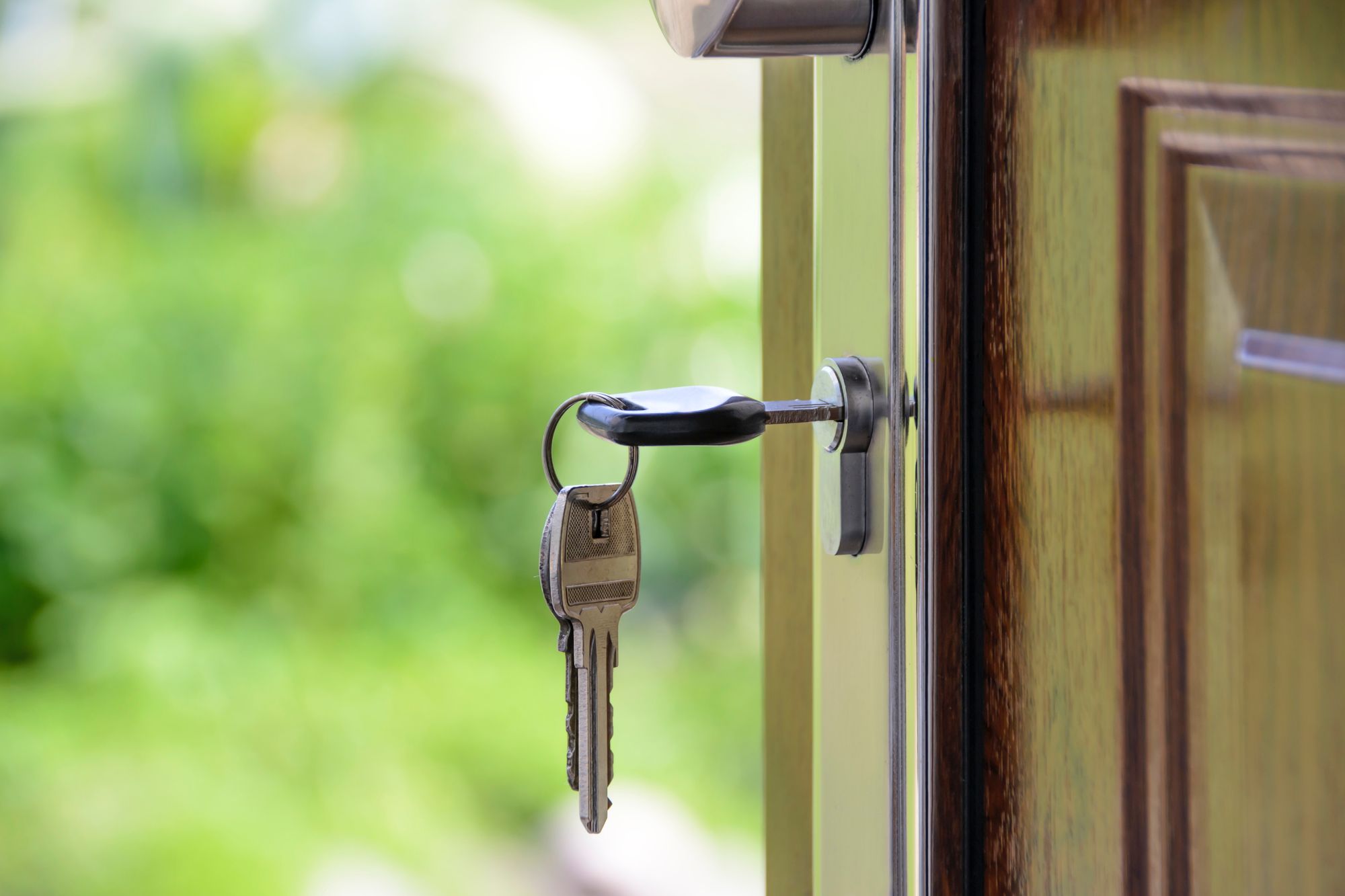
Welcome to the very first instalment of "Byte-Sized tips", where I share solutions to some of the quirkiest challenges I've faced in my coding journey. Here's a real-world solution for a head-scratching issue that you may encounter for those dealing with private keys in environment variables.
We all know that when working with Firebase, credentials are required to access the database. Firebase conveniently provides a JSON file filled with these private goodies, like your project id, private key id, or the private key itself. During local development, it's a breeze to source these from a provided JSON file.
But here's the twist: we all know when we deploy our code, it's bad practice to expose this private data. So, where do we hide it? In the environment variables, of course!
Now, things usually work perfectly locally when we access the environment variables. But when deploying to a platform like Heroku for example and accessing the Heroku vars, a wild error appears:
ValueError: Failed to initialize a certificate credential. Caused by: "No key could be detected."
This error is typically related to special characters in your private key (like \n
) being parsed as literal characters by some shells. So, \n
becomes \\n
.
The usual fix is replacing \\n
with \n
in your code:
os.environ["PRIVATE_KEY"].replace("\\n", "\n")
But sometimes, life doesn't play fair, and this solution doesn't work for everyone. I found myself in such a predicament, even after trying all sorts of variations, including Base64 encoding and decoding the private key.
But, as they say, necessity is the mother of invention. The workaround I discovered involved storing all private values in a dictionary, then saving that dictionary in a single environment variable, so it would look something like this if "TEST_CONFIG" was the name of my environment variable:
TEST_CONFIG:{private_key: "d4b838v", private_key_id:"442224"...etc}
To access the credentials, I would then use:
credentials.Certificate(json.loads(os.environ["TEST_CONFIG"]))
So, instead of keeping each value individually accessible in its environment variable, this bundled approach did the trick for me!
And that, my friends, is our first Byte-Sized Tip. Stay tuned for more and happy coding!